Very useful script to daily
status updates of CPU temperature and attached HDD on your Raspberry
Pi.
To create a script copy the code and make a file with command:
To create a script copy the code and make a file with command:
cd to /path/you/prefered/
sudo nano nameofscript.py #this wiil create blank file and paste code
CODE:
# coding=utf-8
import os
import smtplib
import statvfs
from datetime import timedelta
from email.mime.text import MIMEText
#Read the systems uptime:
with open('/proc/uptime', 'r') as f:
uptime_seconds = float(f.readline().split()[0])
# At First we have to get the current CPU-Temperature with this defined function
def getCPUtemperature():
res = os.popen('vcgencmd measure_temp').readline()
return(res.replace("temp=","").replace("'C\n",""))
# Now we convert our value into a float number
temp = float(getCPUtemperature())
#Read the statistics of the drives in bytes and convert to Gigabytes (using the mount points)
GB = (1024 * 1024) * 1024
HDD = os.statvfs ("/media/SERVICE") # in my case is mounted to /media/ , name is SERVICE
HDD = (HDD.f_frsize * HDD.f_bfree)/GB
#HDD1 = os.statvfs ("/media/HDD2")
#HDD1 = (HDD1.f_frsize * HDD1.f_bfree) / GB
# Enter your smtp Server-Connection
server = smtplib.SMTP('smtp.gmail.com' , 587)
#if your using gmail: smtp.gmail.com
server.ehlo()
server.starttls()
server.ehlo
# Login
server.login("yourmail@gmail.com" , "yourpassword")
# Now comes the Text we want to send. It will send the System Uptime, the CPU Temperature and the free space of your hard drives:
value = "System Uptime (hh:mm:ss) is: " + str(timedelta(seconds = uptime_seconds)) + "\n" + "CPU Temperature is: " + getCPUtemperature() + "C " + "\n" + "HDD Free Space: " + str(HDD) + " GB"
msg = MIMEText(value)
# The Subject of your E-Mail
msg['Subject'] = "Daily Raspberry Pi Status" #you can wtite everyting you prefer
# Consigner of your E-Mail
msg['From'] = "Raspberry Pi"
# recipient of your E-Mail
msg['To'] = "receivermail@gmail.com"
# Finally send the mail
server.sendmail("sendermail@gmail.com", "receivermail@gmail.com", msg.as_string())
server.quit()
You have to change this lines according where your HDD is mounted and name of it:
HDD = os.statvfs ("/media/SERVICE") # in my case is mounted to media and name is SERVICE
HDD = (HDD.f_frsize * HDD.f_bfree)/GB
Check where is maunted and what's the name of HHD with this command:
df -h
You will see it of the bottom of output.
When you are ready with edited code test it with this command:
cd /path/to/script
sudo python nameofscript.py
and wait to receive mail :) If everything is OK add script to crontab:
su - #to become a root
crontab – e # to edit cron jobs
and add this line:
*/*10* * * * python /path/to/yourscript/temp_usbHDD_status.py 2>&1
# with this you will receive mail every day at 10 am.
To save it -> CTRL+O-> Enter
To exit -> CTRL+X
Final result:
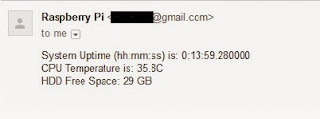
Source
You can buy sensors from HERE